[Re-usable Code] Simple Interactive Chess Engine in Ren'Py
Posted: Wed Jul 18, 2018 11:13 pm
Updates as of 09/16/2018
Updated a bug fix for building Android distribution, thanks to @Imperf3kt and @PyTom.
Updates as of 07/21/2018
A huge thank-you to those who discovered the problem and please accept my apologies!
Updated attachment:
Fatal bug fixes for Player vs. Computer mode. Minor modifications were made in script.rpy and screens.rpy for a smoother exit from the chess game.
The attached code files and files in my github repo have been updated accordingly, and updates to the instructions have been addressed. Please do download this latest version. My apologies for any inconvenience caused!
------
My first post not counting the guestbook!
So I implemented a simple Chess Game in Ren'Py using Screen Language and creator-defined Displayables. I don't know if anyone has done similar things before but if so, please take this as an alternative version! You are more than welcome to incorporate this into any project of yours!
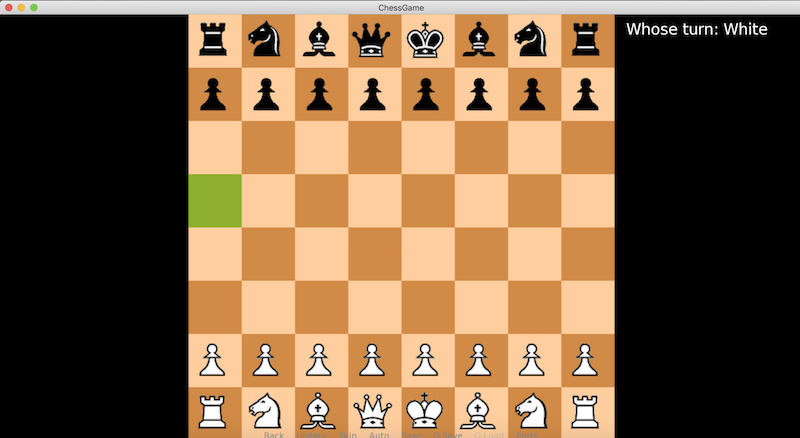
Gameplay and Operations
Click on a piece and all of its available moves will be highlighted. Click on any of the available destination squares to make a move. Press <- on the keyboard to undo moves.
The default window size is 1280 * 720. The Chess Game supports a choice between Player vs. Self and Player vs. Computer.The current version implements the basic chess rules except for castling, en passant and promotion. In Player vs. Computer, the Player plays White by default and the opponent is a minimally-implemented Chess AI.
Feel free to use the code in any project of your choice, free or commercial. Instructions are as follows:
List of core files for the Chess Game:
Paste the following code into specified .rpy files.
In screens.rpy
Note that ai_mode is a Boolean, for Player vs. Self, call screen minigame(False) and for Player vs. Computer, call screen minigame(True)
In script.rpy (or any script file in which the chess game should occur)
Note the way screen minigame() is called with the variable ai_mode
I have included in-text code snippets as well as downloadables at the bottom. You can also find the source code and detailed instructions in my github repo.
And that's it for my first post in Lemma Soft and hope you enjoy it! Thanks for reading this!
Updated a bug fix for building Android distribution, thanks to @Imperf3kt and @PyTom.
Updates as of 07/21/2018
A huge thank-you to those who discovered the problem and please accept my apologies!
Updated attachment:
Fatal bug fixes for Player vs. Computer mode. Minor modifications were made in script.rpy and screens.rpy for a smoother exit from the chess game.
The attached code files and files in my github repo have been updated accordingly, and updates to the instructions have been addressed. Please do download this latest version. My apologies for any inconvenience caused!
------
My first post not counting the guestbook!
So I implemented a simple Chess Game in Ren'Py using Screen Language and creator-defined Displayables. I don't know if anyone has done similar things before but if so, please take this as an alternative version! You are more than welcome to incorporate this into any project of yours!
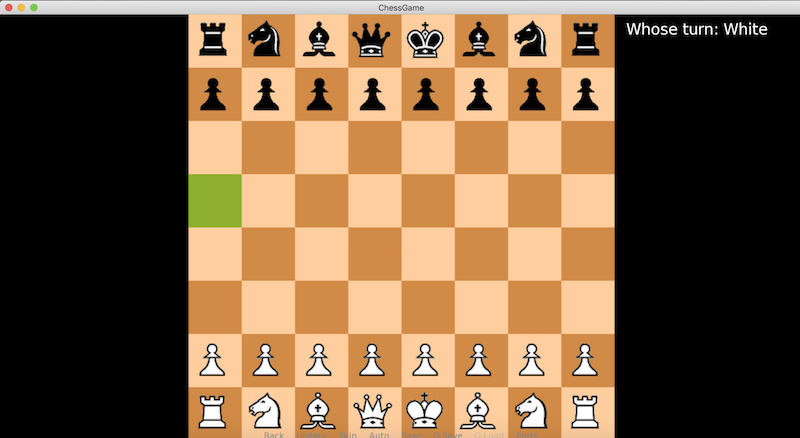
Gameplay and Operations
Click on a piece and all of its available moves will be highlighted. Click on any of the available destination squares to make a move. Press <- on the keyboard to undo moves.
The default window size is 1280 * 720. The Chess Game supports a choice between Player vs. Self and Player vs. Computer.The current version implements the basic chess rules except for castling, en passant and promotion. In Player vs. Computer, the Player plays White by default and the opponent is a minimally-implemented Chess AI.
Feel free to use the code in any project of your choice, free or commercial. Instructions are as follows:
List of core files for the Chess Game:
- images/bg chessboard.png - the chess board image
- images/pieces_image - the chess pieces images
- chesslogic.py - the rules and logic behind chess
- chessai.py - the auto-player that evaluates and selects move
- chessgui.rpy - the Renpy Displayable class and methods
- screens.rpy - the mini-game screen holding the Displayable
- script.rpy - the game script that calls the mini-game screen
Paste the following code into specified .rpy files.
In screens.rpy
Note that ai_mode is a Boolean, for Player vs. Self, call screen minigame(False) and for Player vs. Computer, call screen minigame(True)
Code: Select all
## This screen is used for the chess game.
screen minigame(ai_mode):
default chess = ChessDisplayable(chess_ai=ai_mode)
add "bg chessboard"
add chess
if chess.winner:
timer 6.0 action Return(chess.winner)
In script.rpy (or any script file in which the chess game should occur)
Note the way screen minigame() is called with the variable ai_mode
Code: Select all
label start:
$ ai_mode = False
$ winner = None
"Welcome to the Ren'Py Chess Game!"
label opponent_selection:
menu:
"Please select the game mode."
"Player vs. Self":
$ ai_mode = False
"Player vs. Computer":
$ ai_mode = True
# Player plays White by default
$ player_color = 'White'
$ computer_color = 'Black'
window hide
# Start chess game
call screen minigame(ai_mode)
# End chess game
$ winner = _return
if ai_mode:
if winner == player_color:
"Congratulations! You won!"
elif winner == computer_color:
"The computer defeated you. Better luck next time!"
elif winner == 'draw':
"The game ended in a draw. See if you can win the next time!"
else:
if winner != 'draw':
"The winner is [winner]! Congratulations!"
else:
"The game ended in a draw."
And that's it for my first post in Lemma Soft and hope you enjoy it! Thanks for reading this!